Angular Charts
Using ApexCharts in Angular
ng-ApexCharts is an Angular wrapper component for ApexCharts ready to be integrated into your Angular application to create stunning Charts. In this post, you will learn how to use ng-ApexCharts to create various charts in your web application with ease.Download and Installation
1. Install using npm
npm install apexcharts ng-apexcharts --save
2. Open angular.json and under scripts add:
"scripts": [
"node_modules/apexcharts/dist/apexcharts.min.js"
]
3. Add ng-apexcharts-module to imports
imports: [
BrowserModule,
FormsModule,
ReactiveFormsModule,
NgApexchartsModule,
...
]
Usage
In any component you can use the chart using<apx-chart [series]="series" [chart]="chart" [title]="title"></apx-chart>
You need to provide at least the series and chart attribute to make sure the chart can get created.
Creating your first Angular Chart
Once you have installed the library, you are ready to create a basic bar chart in Angular. So, let’s get started. We start with theapp.component.ts
import { Component, ViewChild } from "@angular/core";
import {
ChartComponent,
ApexAxisChartSeries,
ApexChart,
ApexXAxis,
ApexTitleSubtitle
} from "ng-apexcharts";
export type ChartOptions = {
series: ApexAxisChartSeries;
chart: ApexChart;
xaxis: ApexXAxis;
title: ApexTitleSubtitle;
};
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
@ViewChild("chart") chart: ChartComponent;
public chartOptions: Partial<ChartOptions>;
constructor() {
this.chartOptions = {
series: [
{
name: "My-series",
data: [10, 41, 35, 51, 49, 62, 69, 91, 148]
}
],
chart: {
height: 350,
type: "bar"
},
title: {
text: "My First Angular Chart"
},
xaxis: {
categories: ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep"]
}
};
}
}
And, in app.component.html
, put this
<div style="text-align:center">
<apx-chart
[series]="chartOptions.series"
[chart]="chartOptions.chart"
[xaxis]="chartOptions.xaxis"
[title]="chartOptions.title"
></apx-chart>
</div>
As you can see in the above template, the Angular ApexCharts Component <apx-chart> has 4 attributes. Below, all the other options are listed which can be provided as attributes to the component.
All Options
All options of the ng-chart can be inserted using the attributes. This is a list of all available attributes:
@Input() chart: ApexChart;
@Input() annotations: ApexAnnotations;
@Input() colors: string[];
@Input() dataLabels: ApexDataLabels;
@Input() series: ApexAxisChartSeries | ApexNonAxisChartSeries;
@Input() stroke: ApexStroke;
@Input() labels: string[];
@Input() legend: ApexLegend;
@Input() fill: ApexFill;
@Input() tooltip: ApexTooltip;
@Input() plotOptions: ApexPlotOptions;
@Input() responsive: ApexResponsive[];
@Input() xaxis: ApexXAxis;
@Input() yaxis: ApexYAxis | ApexYAxis[];
@Input() grid: ApexGrid;
@Input() states: ApexStates;
@Input() title: ApexTitleSubtitle;
@Input() subtitle: ApexTitleSubtitle;
@Input() theme: ApexTheme;
This will render the following chart. To read more about the options you can configure in a bar chart, check out this plotOptions.bar configuration.
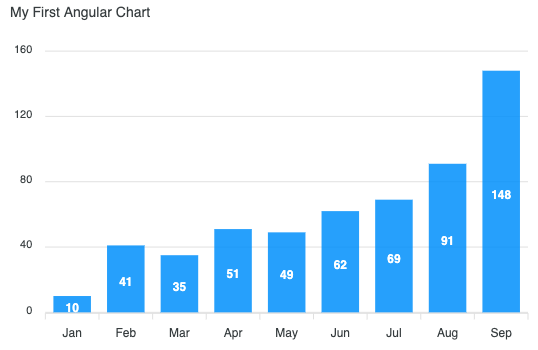
Full Example
The above example can be accessed live at CodeSandbox In the next section, we will see how we can update the rendered chart dynamicallyUpdating Angular Chart Data
Updating your Angular chart data is simple. You just have to update the series attribute and it will automatically trigger event to update the chart. We will see an example below updating the chart data with some random series to illustrate the point.
export class AppComponent {
@ViewChild("chart", { static: false }) chart: ChartComponent;
public chartOptions: Partial<ChartOptions>;
constructor() {
this.chartOptions = {
series: [{
name: 'series-1',
data: [44, 55, 13, 33]
}],
};
}
public updateSeries() {
this.chartOptions.series = [{
data: [23, 44, 1, 22]
}];
}
}
As you can see in the example above, by just changing the props, we trigger the update event of ApexCharts.
More Charts Examples?
There are several other charts that can be created by changing a couple of options. More than 80+ samples can be found on Angular Chart Demos page.Need Advanced Chart Features?
We partnered with Infragistics to give you access to their comprehensive Angular Charts Library. It includes 65+ real-time charts, including Pie Chart, Line Chart, Bar Chart, Donut Chart, Treemap, and even Stock Charts, that provide the same features as the ones you come across with Google Finance and Yahoo Finance Charts. Using Ignite UI for Angular charts when working on your project, you can apply deep analytics, render millions of data points in milliseconds, and provide great UX to end-users.
With many useful Angular chart features like animations, annotations, axis gridlines, chart overlays, chart highlighting, and others, you can build better data-driven, mission-critical web and mobile apps in Angular. The Angular Charts component by Infragistics delivers stunning dashboards and enables you to craft interactive charts and graphs that are designed for speed, functionality, and seamless performance on every modern browser.